티스토리 뷰
1. List (arrayList)를 int 배열로 변경하는 방법
list.stream().mapToInt(Integer::intValue).toArray();
Integer배열을 int로 바꿔주는것은 stream을 이용해야한다.
2. List (arrayList)를 String 배열로 변경하는 방법
// list를 string array로 바꾸는 두가지 방법. 1. stream.toArray(Sting[]::new) 2. list.toArray(new String[0]);
String[] strings = stringList.toArray(new String[0]);
String[] strings2 = stringList.stream().toArray(String[]::new);
위 코드에서 좀 이상한게 보였다. List 인터페이스의 Object toArray(), Object toArray(Object[] a) 메소드에서
지정된 배열인 a 배열에 Collection 객체를 저장해 반환한다고 자바의 정석에는 나와있는데, 왜 String 배열의 size를 0으로 설정해줬는데 되는가?
1. List를 toArray 메서드에 파라메터로 넘어가는 배열 객체의 size만큼의 배열로 전환한다. (위의 예시에서는 size = =0)
2. 단, 해당 List size가 인자로 넘어가는 배열 객체의 size보다 클때, 해당 List의 size로 배열이 만들어진다.
3. 반대로 해당 List size가 인자로 넘어가는 배열객체의 size보다 작을때는, 인자로 넘어가는 배열객체의 size로 배열이 만들어진다.
String[] stringArr = new String[0];
System.out.println("stringArr size :" + stringArr.length );
String[] stringArr2 = new String[10];
System.out.println("stringArr size :" + stringArr.length );
String[] strings = stringList.toArray(stringArr);
String[] strings2 = stringList.toArray(stringArr2);
System.out.println("strings size :" + strings.length);
System.out.println("strings2 size :" + strings2.length);
따라서 위 코드를 실행하면 다음과 같은 결과가 나온다.
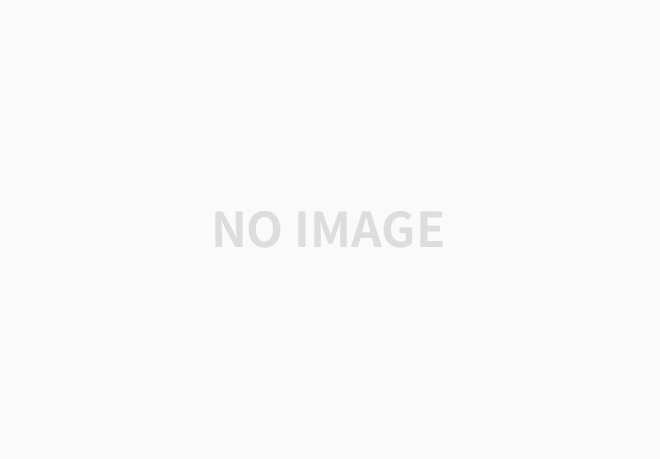
3. list.remove()는 처음 요소 하나만 제거 한다. 모두 제거 하려면 list.removeAll()
반복문 안에서 list.remove같은 함수 쓰면 ConcurrentModificationException 발생.
List<String> list = new ArrayList<>();
list.add("apple");
list.add("kiwi");
list.add("melon");
list.add("banana");
for (String item : list) {
if (item.equals("kiwi")) {
list.remove(item);
}
}
// list.removeIf(item -> item.equals("kiwi"));
위의 코드에서 반복문안에서 list.remove를 하면
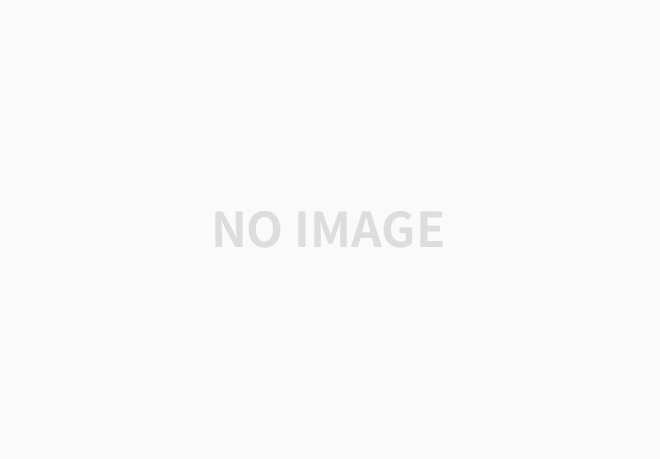
위와 같이 ConcurrentModificationException이라는 Runtime Exception이 발생한다.
왜냐하면 반복문을 통해서 looping 할 때 list의 item을 삭제 했을 때, 다음 참조 하는 item의 index가 list의 범위를 벗어 날 수 있기 때문이다.
또한, ConcurrentModificationException이 발생하지 않더라도, 일부 요소가 탐색에서 누락 될 수 있다.
List<String> list = new ArrayList<>();
list.add("apple");
list.add("kiwi");
list.add("melon");
list.add("banana");
for (int i = 0; i < list.size(); i++) {
String item = list.get(i);
System.out.println("Iterating: " + item);
if (item.equals("apple") || item.equals("kiwi")) {
list.remove(item);
}
}
System.out.println(list);
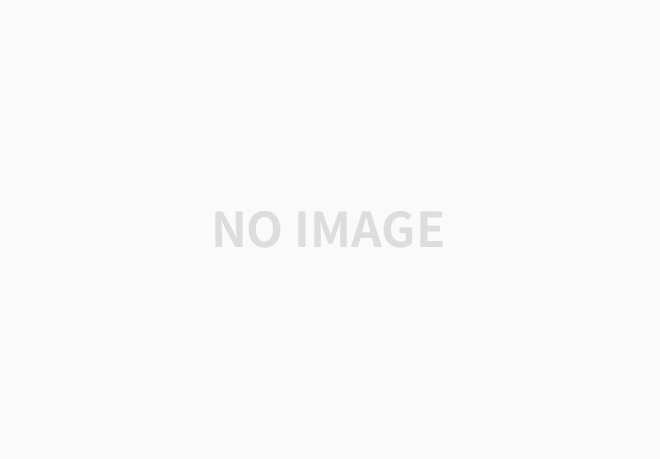
결과값을 보면 kiwi를 삭제하는 것을 누락했다.
apple을 삭제 하면서, index가 변하기 때문에, 1번에 있던 kiwi의 인덱스가 apple이 삭제 되면서 index가 0으로 변하기 때문으로 보인다.
이를 해결 하는 방법은 다음과 같다.
(1) list의 높은 index -> 낮은 index로 돌며 삭제하는방법
: 이러면 index가 밀리는 일이 없어진다.
for (int i = (list.size() - 1); i > -1; i--) {
String item = list.get(i);
System.out.println("Iterating: " + item);
if (item.equals("apple") || item.equals("kiwi")) {
list.remove(item);
}
}
System.out.println(list);
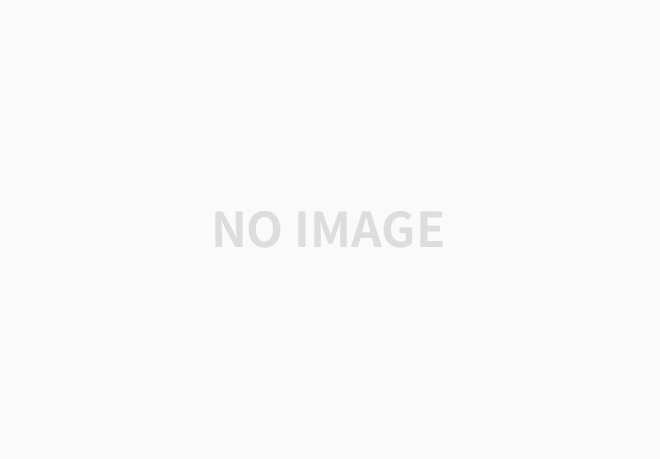
(2) list.removeIf()메소드 사용 하기.
(3) 삭제될 것을 list로 추가 한다음에 removeAll() 메소드로 한번에 삭제.
개인적으로 (2)번이 가장 간결하다.
4. 3개 이상의 값에 대한 최댓값, 최솟값
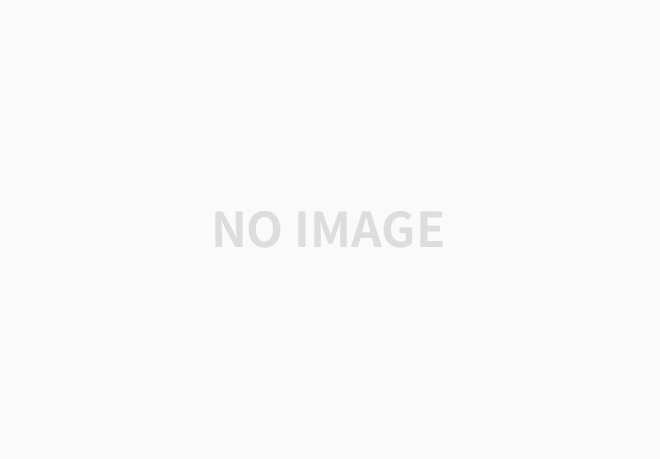
Math.max()에는 매개변수를 2개 밖에 못넣는다.
3개 이상 비교시에서는 Math.max(Math.max(int a, int b),intc) ...로 작성할 수 있다.
5. char 배열의 default 값은 "\0" or "\u0000"
new String(new char[n/2 + 1]).replace("/0","수박").substring(0,n);
// new char[] 통해서 char 배열 생성하면 default 값은 "\0" or "\u0000"임.
위 코드에서 String을 생성할때, 요소를 만들 때, new String[size] 통해서 null을 다루기 보다, char배열로 생성하면 string을 조작 할 수 있다는 것을 배웠다.
공부해야 할 것(확실히 알아야 할것) : LocalDate, Calender 클래스, Comparator과 Comparable에 대해서, 정규식에 대해서. stream 사용시 메소드 들과 FunctionalInterface에 대해서 (람다식) 확실하게.
Reference :
http://asuraiv.blogspot.com/2015/06/java-list-toarray.html
[JAVA] List 의 toArray() 메서드
List 컨테이너의 인스턴스를 배열(array)로 만드는것이 'toArray' 메서드이다. 하지만 이 메서드 사용에 있어서 아래와같은 아리송한 코드를 자주 봤을 것이다. List<String> stringList = new ArrayList<...
asuraiv.blogspot.com
https://codechacha.com/ko/java-remove-from-list-while-iterating/
Java - 반복문 안에서 List의 요소 삭제 방법
List 순환 중에 아이템을 안전하게 삭제하는 방법들을 소개합니다. List 순환(Iterating) 중에 아이템을 삭제하면 ConcurrentModificationException가 발생할 수 있습니다. List의 마지막 Index에서 앞으로 순회, C
codechacha.com
'Programming > Data Structures & Algorithms' 카테고리의 다른 글
이번주 코테문제 풀면서 배운것 3 (0) | 2022.07.19 |
---|---|
이번주 코테문제 풀면서 배운것 2 (0) | 2022.07.17 |
Boyer-Moore 법 (문자열 검색 알고리즘) (0) | 2022.05.28 |
KMP 법 (문자열 검색 알고리즘) (0) | 2022.05.27 |
Brute-Force법 (0) | 2022.05.11 |
- Total
- Today
- Yesterday